Mouse events¶
In the “switch” example we have shown how we can react in a program when the user presses a mouse button. Although for the user a click seems like a single action, we have seen that for the computer it is a sequence of events that starts with an event of the type pg.MOUSEBUTTONDOWN.
In the following examples and tasks, we will use three types of mouse-generated events:
Pressing any mouse button (like in the example with the switch), in which case event.type has a value of pg.MOUSEBUTTONDOWN
Releasing a mouse button, in which case event.type has a value of pg.MOUSEBUTTONUP
Moving the mouse, in which case event.type has a value of pg.MOUSEMOTION. In fact, when moving the mouse multiple such events are generated (each of them describing a small mouse movement in a very short time interval, so each event of this kind usually describes a movement of only a few pixels).
Event objects whose type is pg.MOUSEBUTTONDOWN also contain some additional information, such as:
event.pos - the position of the mouse at the time of registering the event (already used in the switch example)
event.button - a number from 1 to 5 indicating which mouse button is pressed (1 - left, 2 - middle, 3 - right, 4 - scroll up, 5 - scroll down)
Some of the additional event data contained in pg.MOUSEMOTION event objects are:
event.pos - the position of the mouse after the mouse motion event
event.rel - an ordered pair that describes how much the mouse position has changed since the previous mouse motion event
event.buttons - a three-element list of logical values, which determine for each of the three mouse buttons (0 - left, 1 - middle, 2 - right) whether it was pressed during mouse movement.
Click processing - exercises¶
You may not have noticed that in the “switch” program from the previous lesson, the light can be turned on and off by clicking any mouse button. This is because the same type of event is generated for each mouse button, and we did not check which button was pressed when the event occurred.
Task - left button as a switch:
Copy the “switch” program here, then modify it so that the light can be switched on and off only with the left mouse button.
Hint: Use event.button data.
Task - three switches:
Use parts of the “switch” program and create a program that simulates the work of three switches, as shown in the example.
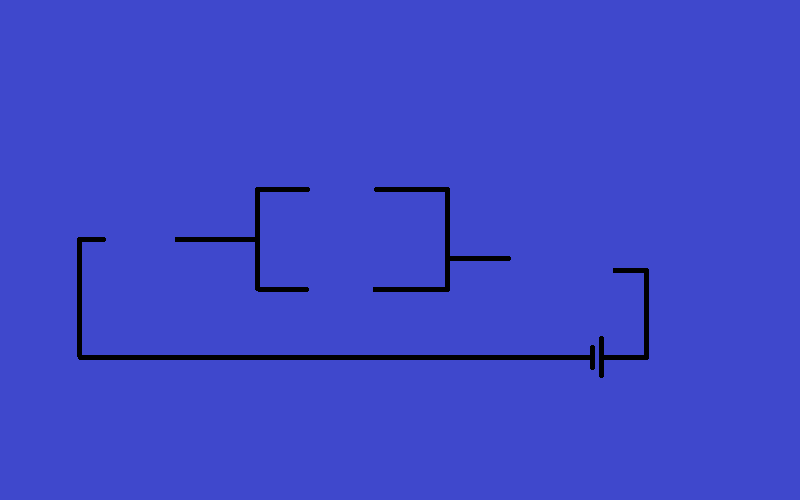
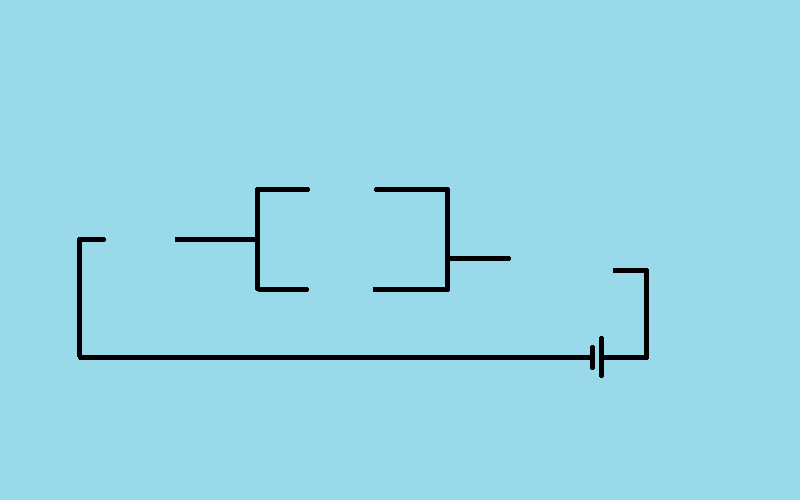


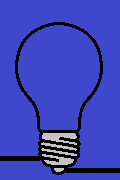
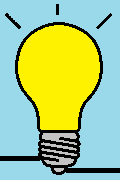
Other mouse events¶
As it was mentioned at the beginning of this lesson, a program can also respond to mouse button release and mouse motion events. To do that, it is necessary to compare the value of event.type with the constants pg.MOUSEBUTTONUP and pg.MOUSEMOTION. The following are tasks where you can try this out.
Task - drawing lines:
Complete the program so that it can draw straight lines, as in the example.
Task - drawing lines with deletion:
Copy the program for drawing lines below, then add an ability to delete all lines with a right-click.
Hint: To distinguish between left and right mouse buttons in the program, the event.button data must be used again. The code in the handle_event function should now look something like this:
Task - dragging:
The following program shows how to allow the user of the program to drag objects.
Try the program out (drag the apples into the basket) and try to understand it, then answer the questions below.

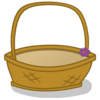
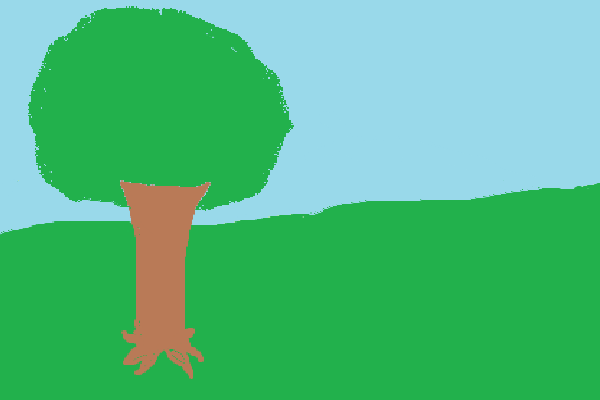
- the index of the apple we are drawing
- Try again
- the index of the apple we are dragging
- Correct
- total number of apples
- Try again
- the number of apples remaining on the tree
- Try again
Q-77: What is the i_apple variable in the program?
-
Q-78: Pair the checks in the program with their meaning.
Try again!
- if mouse_is_on_image(event.pos, basket_pos, basket_image):
- whether the apple should be deleted
- if mouse_is_on_image(event.pos, apple_positions[i]
- whether the user "took" the apple
- if len(apple_positions) == 0:
- whether the game is over
- if i_apple >= 0:
- whether a drag is ongoing
- we read if a mouse button is down during movement
- This is not a convenient way, since the button can be pressed in an empty space (the user did not "take" the object to be dragged)
- dragging is a separate type of event
- No, there is no such type of event
- when plain moving the mouse, the index of the "apple we are dragging" is -1
- Correct
Q-79: How do we distinguish between dragging and plain mouse movement in a program?